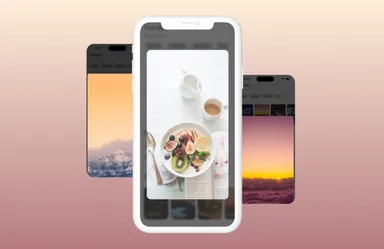
Flutter Dismissible Page
Flutter widget that allows you to dismiss page to any direction, forget the boring back button and plain transitions.
Completed in:
See it live →Flutter widget that allows you to dismiss page to any direction, forget the boring back button and plain transitions.
Features:
- Dismiss to any direction
- Works with nested list view
- Animating border
- Animating background
- Animating scale
Support
PRs Welcome
Discord Channel
Don’t forget to give it a star ⭐
Demo
Live Demo | Multi Direction | Vertical |
---|---|---|
![]() | ![]() | ![]() |
Getting Started
const imageUrl =
'https://user-images.githubusercontent.com/26390946/155666045-aa93bf48-f8e7-407c-bb19-bc247d9e12bd.png';
class FirstPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Color.fromRGBO(228, 217, 236, 1),
body: GestureDetector(
onTap: () {
// Use extension method to use [TransparentRoute]
// This will push page without route background
context.pushTransparentRoute(SecondPage());
},
child: Center(
child: SizedBox(
width: 200,
// Hero widget is needed to animate page transition
child: Hero(
tag: 'Unique tag',
child: Image.network(
imageUrl,
fit: BoxFit.cover,
),
),
),
),
),
);
}
}
class SecondPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return DismissiblePage(
onDismissed: () {
Navigator.of(context).pop();
},
// Note that scrollable widget inside DismissiblePage might limit the functionality
// If scroll direction matches DismissiblePage direction
direction: DismissiblePageDismissDirection.multi,
isFullScreen: false,
child: Hero(
tag: 'Unique tag',
child: Image.network(
imageUrl,
fit: BoxFit.cover,
),
),
);
}
}